Just created my blog for a university assignment. Though I'm wondering how I would implement a delete function in the form of a button. i.e. "click a button to delete a specific blog entry". The blog itself is connected to a database, but I'm not sure how to do so. The following code allows the user to post a comment and also return the list of blog entries. Any ideas?
[syntax=php] <form action=<?php echo "'blog_read.php?pid=".$_GET['pid']."'";?> method="post">
<p>
<label for="user">NAME</label>
<input type="text" name="user" id="user" />
</p>
<p>
<textarea name="body" rows="20" cols="60"></textarea>
<!--<input type="hidden" name="valid_post_id" value="">-->
</p>
<p>
<input type="submit" value="add comment" />
</p>
</form>
<form method="link" action="blog_list.php">
<input type="submit" value="Blog List">
</form>[/syntax]
PHP Delete ...
Re: PHP Delete ...
Well for an actual button you needs to have it as part of a form
[syntax=xhtml]<form action="" method="post">
<div>
<input type="hidden" name="action" value="delete" />
<input type="submit" value="Delete" />
</div>
</form>[/syntax]
The you can check the value of $_POST['action'] to see what the user is trying to do.
A slightly nicer way is to just use a link (which you can style to look like a button if you like)
[syntax=xhtml]<a href="?action=delete" title="Delete Post">Delete</a>[/syntax]
For this you would need to check $_GET['action']. So that is the UI stuff (I'll leave working out passing the posts ID to you)
The next thing would be to make a function to delete a post, just a simple DELETE query.
[syntax=php]function delete_post($post_id){
$post_id = (int)$post_id;
mysql_query("DELETE FROM `posts` WHERE `post_id` = {$post_id}");
mysql_query("DELETE FROM `comments` WHERE `post_id` = {$post_id}");
}[/syntax]
Not much you can do about having to do two queries here.
So now you just need to be able to use this function on the page, which is essentially the same as you did for the add comment bit.
[syntax=php]if (isset($_POST['action']) && $_POST['action'] === 'delete'){
$errors = array();
if (bad_thing){
$errors[] = ':(';
}
if (empty($errorrs)){
delete_post($_GET['post_id']);
}
}[/syntax]
Make sense ?
[syntax=xhtml]<form action="" method="post">
<div>
<input type="hidden" name="action" value="delete" />
<input type="submit" value="Delete" />
</div>
</form>[/syntax]
The you can check the value of $_POST['action'] to see what the user is trying to do.
A slightly nicer way is to just use a link (which you can style to look like a button if you like)
[syntax=xhtml]<a href="?action=delete" title="Delete Post">Delete</a>[/syntax]
For this you would need to check $_GET['action']. So that is the UI stuff (I'll leave working out passing the posts ID to you)
The next thing would be to make a function to delete a post, just a simple DELETE query.
[syntax=php]function delete_post($post_id){
$post_id = (int)$post_id;
mysql_query("DELETE FROM `posts` WHERE `post_id` = {$post_id}");
mysql_query("DELETE FROM `comments` WHERE `post_id` = {$post_id}");
}[/syntax]
Not much you can do about having to do two queries here.
So now you just need to be able to use this function on the page, which is essentially the same as you did for the add comment bit.
[syntax=php]if (isset($_POST['action']) && $_POST['action'] === 'delete'){
$errors = array();
if (bad_thing){
$errors[] = ':(';
}
if (empty($errorrs)){
delete_post($_GET['post_id']);
}
}[/syntax]
Make sense ?
Re: PHP Delete ...
Ah thank you so much! It makes perfect sense, and will certainly buff up my marks - I'll be sure to reference you and your website.
Thanks jacek
Thanks jacek

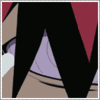
Re: PHP Delete ...
Noso1066 wrote: I'll be sure to reference you and your website.
*thumbs up icon that is missing from this forum for some reason*
Noso1066 wrote:Thanks jacek
No problem
